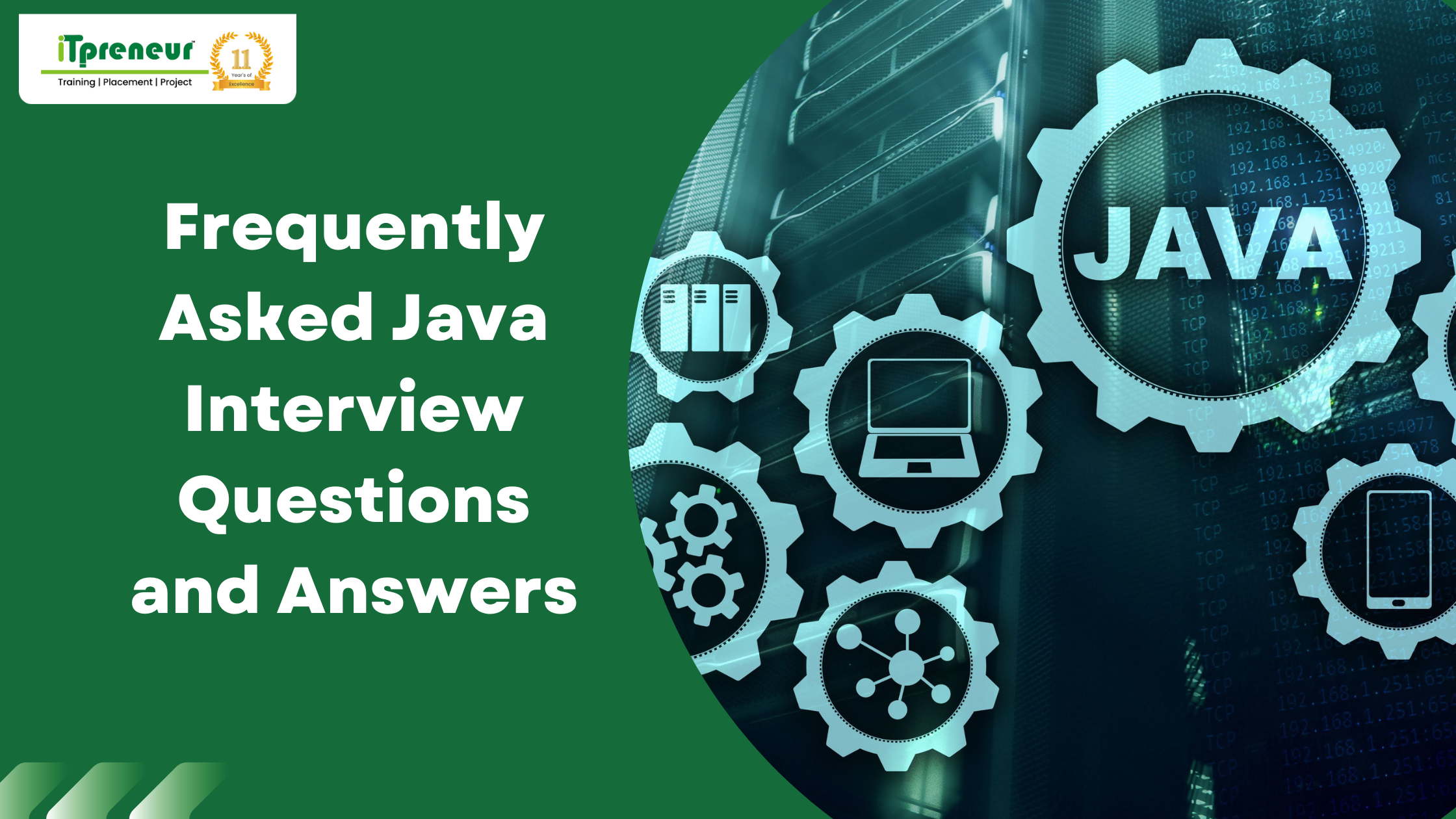
Frequently Asked Java Interview Questions and Answers
Feeling nervous about your Java interview? Don’t sweat it! This blog equips you with the most frequently asked Java interview questions and answers, giving you the confidence to ace your coding challenge.
Introduction
The landscape of software development is brimming with opportunity, and Java remains a cornerstone technology for countless businesses. This comprehensive blog post equips you with the knowledge and tools to conquer your Java interview. We’ve compiled a treasure trove of frequently asked interview questions, meticulously categorized for your ease of reference. From core Java concepts to advanced functionalities, consider this your one-stop shop for interview domination.
Java Fundamentals
Before we take a dive into the intricate and interesting world of Java interviews, let’s strengthen the foundation – here are some questions and their answers on the fundamentals of Java.
Q1. What is Java and Why is it Popular?
Java is a high-level, general-purpose programming language known for its:
- Simplicity: Java syntax is relatively easy to learn and understand, making it a good starting point for new programmers.
- Object-Oriented Programming (OOP): Java heavily utilizes OOP principles, which helps organize code into reusable and maintainable modules.
- Platform Independence: Java code is compiled into bytecode, which can run on any machine with a Java Virtual Machine (JVM) installed. This allows developers to “write once, run anywhere.”
- Robust Security: Java enforces certain security measures by default, helping to prevent common coding vulnerabilities.
These features make Java a versatile and popular choice for developing a wide range of applications, from web and mobile apps to enterprise software and even embedded systems.
Q2. Explain JDK, JRE, and JVM
Think of developing a photo:
- JDK (Java Development Kit): This is your complete development toolkit. It includes a compiler that translates your Java code into bytecode, a debugger to help identify and fix errors, and various libraries containing pre-written code for common functionalities.
- JRE (Java Runtime Environment): This acts like a photo viewer. It allows you to run Java programs on your computer. The JRE includes the JVM and essential class libraries needed to interpret and execute the bytecode generated by the compiler.
- JVM (Java Virtual Machine): This is the software that interprets the bytecode instructions. It’s like a universal translator that allows Java bytecode to run on any machine with a JVM installed, regardless of the underlying operating system.
Q3. What are the Pillars of OOP?
Object-oriented programming is a fundamental paradigm in Java. Let’s understand it with a house-building analogy:
- Encapsulation: Imagine keeping the blueprints (data) and construction plans (methods) of your house together within a single unit called a class. This promotes data protection and code organization.
- Inheritance: Building a smaller cottage (subclass) based on the blueprint (superclass) of a larger house allows you to reuse existing functionalities while adding specific features for the cottage.
- Polymorphism: The ability of different objects (houses) of the same class (house design) to respond differently to the same message (method call) based on their specific type. Think of different colored houses responding to “paint” differently.
- Abstraction: Focusing on the essential features of a house (living space, bedrooms) and hiding the complex details of plumbing and wiring allows for cleaner and easier code management.
Q4. Classes vs. Objects
- A class is a blueprint or template that defines the properties (variables like color, model) and functionalities (methods like startEngine(), accelerate()) of similar objects. It’s like a detailed plan for building a house.
- An object is an instance of a class. It’s an actual house built from the blueprint. There can be many individual houses (objects) of the same design (class). For example, a Car class might have properties like color and model, and methods like startEngine() and accelerate(). Each car (e.g., your red Honda Civic) would be an object created from the Car class.
Example: You can define a Car class with properties like color, model, and methods like startEngine() and accelerate(). Each individual car (e.g., your red Honda Civic) would be an object created from the Car class.
Q5.What are the different Data Types in Java ?
Java has two main categories of data types for storing information:
- Primitive Data Types: These are basic building blocks to store simple values like integers (int), floating-point numbers (double), characters (char), and boolean values (true/false).
- Reference Data Types: These store references (memory addresses) to complex objects like arrays, strings, and custom classes. Think of them as pointers to the actual data stored elsewhere in memory.
- Functionality based questions
Q6. Explain the difference between a for loop and a while loop. When would you use each?
- For loops excel at iterating a specific number of times. They provide a clear structure with an initialization step (setting up a counter), a condition that determines how long the loop runs, and an update step (usually incrementing the counter) that moves the loop forward. It’s like having a recipe with exact measurements and steps for a set number of repetitions.
- While loops offer more flexibility. They continue executing as long as a certain condition remains true. This is useful when you don’t know the exact number of iterations beforehand. Think of it like a recipe that instructs you to keep adding ingredients until you achieve a specific consistency.
- For loops: When you know the exact number of repetitions upfront.
- While loops: When you need to loop based on a changing condition.
Q7. What is an array in Java? How do you declare and initialize an array?
Imagine a filing cabinet with labeled drawers. An array is similar, but it stores elements of the same data type in contiguous memory locations. This makes accessing and manipulating elements efficient. Declaring an array involves specifying the data type it can hold and the number of elements it can store. You can then initialize the array with specific values or leave it empty for later assignment.
Q8. Explain the concept of method overloading with an example.
Method overloading allows you to create multiple methods in a class with the same name, but they must be differentiated by their parameter lists. This provides flexibility in how you call the method based on the data you want to use. Think of it like having a tool with different attachments. The core tool (method name) remains the same, but the attachments (parameters) change its functionality for specific tasks.
Questions on Advanced Java Concepts
Q9. Explain the difference between final, finally, and finalize keywords in Java.
final:
- A modifier used with variables, methods, and classes.
- For variables: Makes the value constant after initialization, preventing further changes. (Think of a locked box where you can’t replace the contents)
- For methods: Prevents subclasses from overriding the method, enforcing specific behavior. (Think of a sealed recipe that can’t be altered)
- For classes: Prevents inheritance from the class, ensuring its functionality remains unchanged. (Think of a foundation that can’t be built upon)
finally:
- A block used within a try-catch block.
- Always executes regardless of whether an exception is thrown or not within the try block. (Think of a safety net that activates no matter what)
- Commonly used for resource cleanup (closing files, database connections) to ensure they’re released properly.
finalize:
- A method declared in the Object class.
- May be called by the garbage collector just before an object is reclaimed. (Think of a final call before an object is removed)
- Generally discouraged due to unpredictable timing and potential for errors. Modern Java encourages using try-with-resources for resource management.
Q10. Describe the concept of garbage collection in Java and its importance.
Java features automatic garbage collection, a crucial mechanism for memory management. Here’s why it’s important:
- Prevents memory leaks: Without garbage collection, you’d be responsible for manually freeing memory used by objects that are no longer needed. This can lead to memory leaks, where unused objects remain allocated, causing performance degradation.
- Simplifies development: With garbage collection, you don’t need to worry about memory management specifics. It frees you to focus on the core logic of your program.
- Improves memory efficiency: The garbage collector identifies unused objects and reclaims their memory for new objects. This ensures efficient memory utilization.
In essence, garbage collection automates memory management in Java, making it easier and safer to develop applications.
Conclusion
You’ve trained, you’ve prepped, and now you’re a Java interview warrior. Remember: strong fundamentals, a dash of advanced knowledge, and consistent practice are your weapons. Conquer your interview with confidence, and land your dream developer job!